A Primer On Scientific Programming With Python 3rd Pdf Merge
- Game Programming With Python
- Learning Scientific Programming With Python Pdf
- Introduction To Computation And Programming Using Python 3rd Edition Pdf
Is it possible, using Python, to merge separate PDF files?
Programming and Scientific Computing in for Aerospace Engineers AE Tutorial Programming Python v3.11 Jacco Hoekstra 0 10 20 30 40 50 60 70 5 0 5 10 15 20 25 30 35 40. 2 print “Hello world!” Hello world! If languagepython: programming = fun. 3 Table of Contents 1. This repository contains resources for the Springer book. A Primer on Scientific Programming with Python, by H. Langtangen, from the 3rd edition and onwards. See index.html for contents. The book refers to this file as the official web page for resources associated with the book.
Assuming so, I need to extend this a little further. I am hoping to loop through folders in a directory and repeat this procedure.
And I may be pushing my luck, but is it possible to exclude a page that is contained in of the PDFs (my report generation always creates an extra blank page).
Btibert3Btibert39 Answers
Use Pypdf or its successor PyPDF2:
A Pure-Python library built as a PDF toolkit. It is capable of:
* splitting documents page by page,
* merging documents page by page,
(and much more)
Here's a sample program that works with both versions.
GillesGillesYou can use PyPdf2s PdfMerger
class.
File Concatenation
You can simply concatenate files by using the append
method.
You can pass file handles instead file paths if you want.
File Merging
If you want more fine grained control of merging there is a merge
method of the PdfMerger
, which allows you to specify an insertion point in the output file, meaning you can insert the pages at any place in the file. The append
method can be thought of as a merge
where the insertion point is the end of the file.

e.g.
Here we insert the whole pdf into the output but at page 2.
Page Ranges
If you wish to control which pages are appended from a particular file, you can use the pages
keyword argument of append
and merge
, passing a tuple in the form (start, stop[, step])
(like the regular range
function).
Game Programming With Python
e.g.
If you specify an invalid range you will get an IndexError
.
Note: also that to avoid files being left open, the PdfFileMerger
s close method should be called when the merged file has been written. This ensures all files are closed (input and output) in a timely manner. It's a shame that PdfFileMerger
isn't implemented as a context manager, so we can use the with
keyword, avoid the explicit close call and get some easy exception safety.
You might also want to look at the pdfcat
script provided as part of pypdf2. You can potentially avoid the need to write code altogether.
The PyPdf2 github also includes some example code demonstrating merging. Expert choice v11 exercise.
Paul RooneyPaul RooneyIs it possible, using Python, to merge seperate PDF files?
Yes.
The following example merges all files in one folder to a single new PDF file:
Martin ThomaMartin ThomaThe pdfrw
library can do this quite easily, assuming you don't need to preserve bookmarks and annotations, and your PDFs aren't encrypted. cat.py
is an example concatenation script, and subset.py
is an example page subsetting script.
The relevant part of the concatenation script -- assumes inputs
is a list of input filenames, and outfn
is an output file name:
As you can see from this, it would be pretty easy to leave out the last page, e.g. something like:
Disclaimer: I am the primary pdfrw
author.
Put the pdf files in a dir. Launch the program. You get one pdf with all the pdfs merged.
Giovanni PythonGiovanni PythonLearning Scientific Programming With Python Pdf
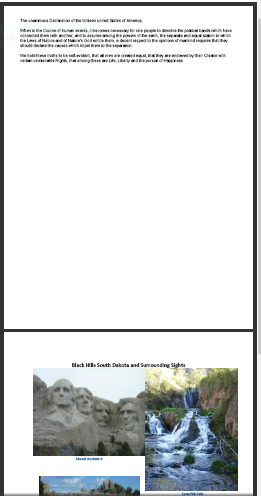
here, http://pieceofpy.com/2009/03/05/concatenating-pdf-with-python/, gives an solution.
Introduction To Computation And Programming Using Python 3rd Edition Pdf
similarly:
Mark KMark KGit Repo: https://github.com/mahaguru24/Python_Merge_PDF.git
A slight variation using a dictionary for greater flexibility (e.g. sort, dedup):
It’s also possible to use Aspose.PDF Cloud SDK for Python.Here is a quick example:
The biggest benefit is that API provides many other possibilities to manage your PDFs. You can modify, convert, encrypt files; handle pages, text, shapes and other elements.
Note: I am working as Developer Evangelist at Aspose.
protected by Community♦Jan 18 '18 at 5:34
Thank you for your interest in this question. Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?